|
1、文件操作相关函数
1 stat/lstat函数
- 函数描述: 获取文件属性
- 函数原型:
- int stat(const char *pathname, struct stat *buf);
- int lstat(const char *pathname, struct stat *buf);
- 函数返回值: 成功返回0; 失败返回-1
struct stat {
dev_t st_dev; //文件的设备编号
ino_t st_ino; //节点
mode_t st_mode; //文件的类型和存取的权限
nlink_t st_nlink; //连到该文件的硬连接数目,刚建立的文件值为1
uid_t st_uid; //用户ID
gid_t st_gid; //组ID
dev_t st_rdev; //(设备类型)若此文件为设备文件,则为其设备编号
off_t st_size; //文件字节数(文件大小)
blksize_t st_blksize; //块大小(文件系统的I/O 缓冲区大小)
blkcnt_t st_blocks; //块数
time_t st_atime; //最后一次访问时间
time_t st_mtime; //最后一次修改时间
time_t st_ctime; //最后一次改变时间(指属性)
};
- st_mode -- 16位整数 (上面结构体的一个成员)
○ 0-2 bit -- 其他人权限
S_IROTH 00004 读权限
S_IWOTH 00002 写权限
S_IXOTH 00001 执行权限
S_IRWXO 00007 掩码, 过滤 st_mode中除其他人权限以外的信息
○ 3-5 bit -- 所属组权限
S_IRGRP 00040 读权限
S_IWGRP 00020 写权限
S_IXGRP 00010 执行权限
S_IRWXG 00070 掩码, 过滤 st_mode中除所属组权限以外的信息
○ 6-8 bit -- 文件所有者权限
S_IRUSR 00400 读权限
S_IWUSR 00200 写权限
S_IXUSR 00100 执行权限
S_IRWXU 00700 掩码, 过滤 st_mode中除文件所有者权限以外的信息
- If (st_mode & S_IRUSR) -----为真表明可读
- If (st_mode & S_IWUSR) ------为真表明可写
- If (st_mode & S_IXUSR) ------为真表明可执行

S_IFSOCK 0140000 套接字
S_IFLNK 0120000 符号链接(软链接)
S_IFREG 0100000 普通文件
S_IFBLK 0060000 块设备
S_IFDIR 0040000 目录
S_IFCHR 0020000 字符设备
S_IFIFO 0010000 管道
S_IFMT 0170000 掩码,过滤 st_mode中除文件类型以外的信息
- If ((st_mode & S_IFMT)==S_IFREG) ----为真普通文件 等价于: if(S_ISREG(st_mode)) ------为真表示普通文件
- if(S_ISDIR(st.st_mode)) ------为真表示目录文件
上述内容可以通过 man 2 stat查到,用的时候不记得只要查一查,示例都有:
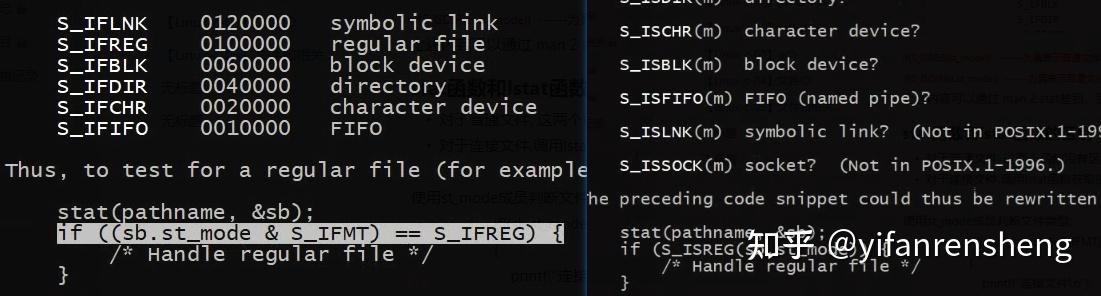
stat函数和lstat函数的区别
- 对于普通文件, 这两个函数没有区别, 是一样的.
- 对于连接文件,调用lstat函数获取的是链接文件本身的属性信息; 而stat函数获取的是链接文件指向的文件的属性信息.
实验1:stat函数测试: 获取文件大小, 文件属主和组
stat.c
//stat函数测试: 获取文件大小, 文件属主和组
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
//int stat(const char *pathname, struct stat *buf);
struct stat st;
stat(argv[1], &st);
printf(&#34;size:[%d], uid:[%d], gid:[%d]\n&#34;, st.st_size, st.st_uid, st.st_gid);
return 0;
}结果:
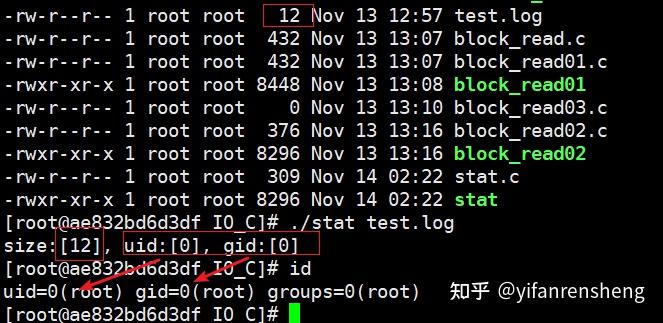
实验2:判断文件类型及权限
//stat函数测试: 获取文件类型和权限
int main(int argc, char *argv[])
{
//int stat(const char *pathname, struct stat *buf);
//获取文件属性
struct stat sb;
stat(argv[1], &sb);
//获取文件类型
if ((sb.st_mode & S_IFMT) == S_IFREG)
{
printf(&#34;普通文件\n&#34;);
}
else if((sb.st_mode & S_IFMT) ==S_IFDIR)
{
printf(&#34;目录文件\n&#34;);
}
else if((sb.st_mode & S_IFMT) ==S_IFLNK)
{
printf(&#34;连接文件\n&#34;);
}
//获取文件类型 另一种形式
if (S_ISREG(sb.st_mode))
{
printf(&#34;普通文件\n&#34;);
}
else if(S_ISDIR(sb.st_mode))
{
printf(&#34;目录文件\n&#34;);
}
else if(S_ISLNK(sb.st_mode))
{
printf(&#34;连接文件\n&#34;);
}
//判断文件权限
if(sb.st_mode & S_IROTH)
{
printf(&#34;---R----&#34;);
}
if(sb.st_mode & S_IWOTH)
{
printf(&#34;---W----&#34;);
}
if(sb.st_mode & S_IXOTH)
{
printf(&#34;---X----&#34;);
}
printf(&#34;\n&#34;);
return 0;
}
结果:
[root@ae832bd6d3df IO_C]# ln -s test.log test.log.ls
[root@ae832bd6d3df IO_C]# ls -ltr
-rw-r--r-- 1 root root 13 Nov 14 03:10 test.log
lrwxrwxrwx 1 root root 8 Nov 14 03:11 test.log.ls -> test.log
[root@ae832bd6d3df IO_C]#
[root@ae832bd6d3df IO_C]# ./stat02 test.log.s
[root@ae832bd6d3df IO_C]# ./stat02 test.log.ls
普通文件
普通文件
---R----
[root@ae832bd6d3df IO_C]# ./stat02 test.log
普通文件
普通文件
---R----
[root@ae832bd6d3df IO_C]# chmod o+w test.log
[root@ae832bd6d3df IO_C]# ./stat02 test.log
普通文件
普通文件
---R-------W----注意:这里使用stat对链接文件无法检测出来;stat(argv[1], &sb); 将代码stat修改为:lstat(argv[1], &sb); 则得到下面的形式:
[root@ae832bd6d3df IO_C]# ./lstat02 test.log.ls
连接文件
连接文件
---R-------W-------X----
[root@ae832bd6d3df IO_C]# ./lstat02 test.log
普通文件
普通文件
---R-------W----同样地,对1的实例进行同样的修改:得到的结果也稍微不同:
[root@ae832bd6d3df IO_C]# ./lstat test.log.ls
size:[8], uid:[0], gid:[0]
[root@ae832bd6d3df IO_C]# ./lstat test.log
size:[13], uid:[0], gid:[0]2、目录操作相关函数
1 opendir readdir closedir
opendir函数
- 函数描述:打开一个目录
- 函数原型: DIR *opendir(const char *name);
- 函数返回值: 指向目录的指针
- 函数参数: 要遍历的目录(相对路径或者绝对路径)
readdir函数
- 函数描述: 读取目录内容--目录项
- 函数原型: struct dirent *readdir(DIR *dirp);
- 函数返回值: 读取的目录项指针
- 函数参数: opendir函数的返回值
struct dirent
{
ino_t d_ino; // 此目录进入点的inode
off_t d_off; // 目录文件开头至此目录进入点的位移
signed short int d_reclen; // d_name 的长度, 不包含NULL 字符
unsigned char d_type; // d_name 所指的文件类型
char d_name[256]; // 文件名
};
d_type的取值:
DT_BLK - 块设备
DT_CHR - 字符设备
DT_DIR - 目录
DT_LNK - 软连接
DT_FIFO - 管道
DT_REG - 普通文件
DT_SOCK - 套接字
DT_UNKNOWN - 未知closedir函数
- 函数描述: 关闭目录
- 函数原型: int closedir(DIR *dirp);
- 函数返回值: 成功返回0, 失败返回-1
- 函数参数: opendir函数的返回值
2 实验 读取目录内容的一般步骤
- DIR *pDir = opendir(“dir”); //打开目录
- while((p=readdir(pDir))!=NULL){} //循环读取文件
- closedir(pDir); //关闭目录
opendir.h
//目录操作测试: opendir readdir closedir
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
#include <dirent.h>
int main(int argc, char *argv[])
{
//打开目录
//DIR *opendir(const char *name);
DIR *pDir = opendir(argv[1]);
if(pDir==NULL)
{
perror(&#34;opendir error&#34;);
return -1;
}
//循环读取目录项
//struct dirent *readdir(DIR *dirp);
struct dirent *pDent = NULL;
while((pDent=readdir(pDir))!=NULL)
{
//过滤掉.和..文件
if(strcmp(pDent->d_name, &#34;.&#34;)==0 || strcmp(pDent->d_name, &#34;..&#34;)==0)
{
continue;
}
printf(&#34;[%s]---->&#34;, pDent->d_name);
//判断文件类型
switch(pDent->d_type)
{
case DT_REG:
printf(&#34;普通文件&#34;);
break;
case DT_DIR:
printf(&#34;目录文件&#34;);
break;
case DT_LNK:
printf(&#34;链接文件&#34;);
break;
default:
printf(&#34;未知文件&#34;);
}
printf(&#34;\n&#34;);
}
//关闭目录
closedir(pDir);
return 0;
}
结果:
[test.log.ls]---->链接文件
[lstat02.c]---->普通文件
[lstat02]---->普通文件
[lstat.c]---->普通文件
[lstat]---->普通文件
[opendir.c]---->普通文件
[opendir]---->普通文件3、dup/dup2/fcntl
1 dup函数
- 函数描述: 复制文件描述符
- 函数原型: int dup(int oldfd);
- 函数参数: oldfd -要复制的文件描述符
- 函数返回值:
- 成功: 返回最小且没被占用的文件描述符
- 失败: 返回-1, 设置errno值
实验:编写程序, 测试dup函数
dup.c
//测试dup函数复制文件描述符
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
//打开文件
int fd = open(argv[1], O_RDWR);
if(fd<0)
{
perror(&#34;open error&#34;);
return -1;
}
//调用dup函数复制fd
int newfd = dup(fd);
printf(&#34;newfd:[%d], fd:[%d]\n&#34;, newfd, fd);
//使用fd对文件进行写操作
write(fd, &#34;hello world&#34;, strlen(&#34;hello world&#34;));
//调用lseek函数移动文件指针到开始处
lseek(fd, 0, SEEK_SET);
//使用newfd读文件
char buf[64];
memset(buf, 0x00, sizeof(buf));
int n = read(newfd, buf, sizeof(buf));
printf(&#34;read over: n==[%d], buf==[%s]\n&#34;, n, buf);
//关闭文件
close(fd);
close(newfd);
return 0;
}
结果:
[root@ae832bd6d3df IO_C]# ./dup test.log
newfd:[4], fd:[3]
read over: n==[13], buf==[hello world!
]
2 dup2函数
- 函数描述: 复制文件描述符
- 函数原型: int dup2(int oldfd, int newfd);
- 函数参数:
- oldfd-原来的文件描述符
- newfd-复制成的新的文件描述符
- 函数返回值:
- 成功: 将oldfd复制给newfd, 两个文件描述符指向同一个文件
- 失败: 返回-1, 设置errno值
0假设newfd已经指向了一个文件,首先close原来打开的文件,然后newfd指向oldfd指向的文件。若newfd没有被占用,newfd指向oldfd指向的文件.
实验 测试dup2函数实现文件描述符的复制
//测试dup2函数复制文件描述符
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
//打开文件
int oldfd = open(argv[1], O_RDWR | O_CREAT, 0755);
if(oldfd<0)
{
perror(&#34;open error&#34;);
return -1;
}
int newfd = open(argv[2], O_RDWR | O_CREAT, 0755);
if(newfd<0)
{
perror(&#34;open error&#34;);
return -1;
}
//调用dup2函数复制fd 使用这个函数后,会让newfd指向oldfd,所以最后argv[2]的文件会是空的
dup2(oldfd, newfd);
printf(&#34;newfd:[%d], oldfd:[%d]\n&#34;, newfd, oldfd);
//使用newfd对文件进行写操作
write(newfd, &#34;hello world&#34;, strlen(&#34;hello world&#34;));
//调用lseek函数移动文件指针到开始处
lseek(newfd, 0, SEEK_SET);
//使用newfd读文件
char buf[64];
memset(buf, 0x00, sizeof(buf));
int n = read(oldfd, buf, sizeof(buf));
printf(&#34;read over: n==[%d], buf==[%s]\n&#34;, n, buf);
//关闭文件
close(oldfd);
close(newfd);
return 0;
}
结果:
[root@ae832bd6d3df IO_C]# make dup2
cc dup2.c -o dup2
[root@ae832bd6d3df IO_C]# ./dup2 tmp1.log tmp2.log
newfd:[4], oldfd:[3]
read over: n==[11], buf==[hello world]
[root@ae832bd6d3df IO_C]# ls -lht
total 220K
-rwxr-xr-x 1 root root 11 Nov 14 06:43 tmp1.log
-rwxr-xr-x 1 root root 0 Nov 14 06:43 tmp2.log
-rwxr-xr-x 1 root root 8.7K Nov 14 06:42 dup2结论:
- make dup2也可已经编译;
- tmp2.log为0,符合预期,因为使用dup2(oldfd, newfd); 函数后,会让newfd指向oldfd,所以最后argv[2]的文件会是空的
- dup2(oldfd, newfd) 记忆的时候可以参考 cp tmp1.log tmp.log 表示后面的跟着前面的内容走;
实验:dup2 完成终端标准输出重定向到文件中
dup2_1.c
//使用dup2函数实现标准输出重定向操作
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
printf(&#34;test1 ....&#34;);
//打开文件
int fd = open(argv[1], O_RDWR | O_CREAT, 0777);
if(fd<0)
{
perror(&#34;open error&#34;);
return -1;
}
printf(&#34;test ....&#34;);
//调用dup2函数实现文件重定向操作
dup2(fd, STDOUT_FILENO);
printf(&#34;ni hao hello world&#34;);
close(fd);
//close(STDOUT_FILENO);
return 0;
}结果:
[root@ae832bd6d3df IO_C]# cat hello.log
test1 ....test ....ni hao hello world说明:
- //close(STDOUT_FILENO);要注释掉,否则无法写入到后面的log中;
- 无论printf() 在dup2() 前还是后,都会被写入进去。
3 dup和dup2小结
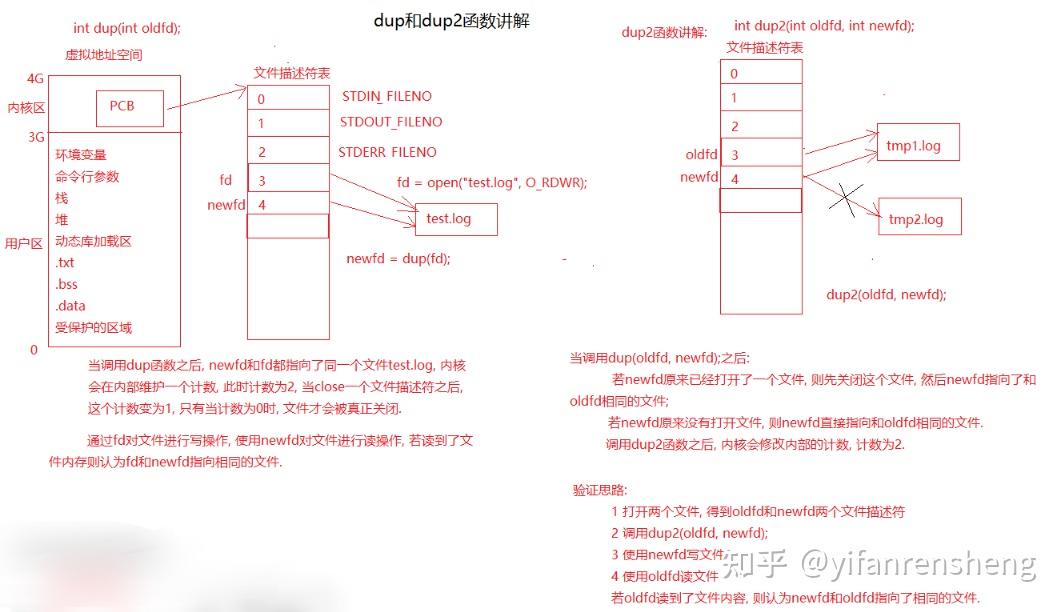
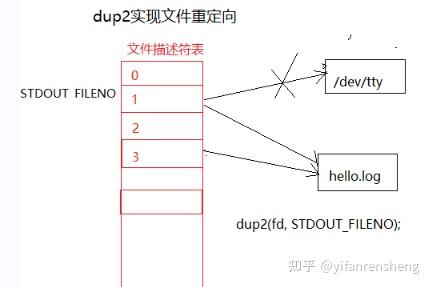
4 fcntl函数
函数描述: 改变已经打开的文件的属性
函数原型: int fcntl(int fd, int cmd, ... /* arg */ );
- 若cmd为F_DUPFD, 复制文件描述符, 与dup相同
- 若cmd为F_GETFL, 获取文件描述符的flag属性值
- 若cmd为 F_SETFL, 设置文件描述符的flag属性
函数返回值:返回值取决于cmd
- 成功
- 若cmd为F_DUPFD, 返回一个新的文件描述符
- 若cmd为F_GETFL, 返回文件描述符的flags值
- 若cmd为 F_SETFL, 返回0
- 失败返回-1, 并设置errno值.
fcntl函数常用的操作:
- 复制一个新的文件描述符:int newfd = fcntl(fd, F_DUPFD, 0); 这个和dup功能完全一样。
- 获取文件的属性标志 int flag = fcntl(fd, F_GETFL, 0)
- 设置文件状态标志
- flag = flag | O_APPEND;
- fcntl(fd, F_SETFL, flag)
- 常用的属性标志
- O_APPEND-----设置文件打开为末尾添加
- O_NONBLOCK-----设置打开的文件描述符为非阻塞
实验1 使用fcntl函数实现复制文件描述符
将3的第一个实验的一条语句改下即可:int newfd = dup(fd) ---> int newfd = fcntl(fd, F_DUPFD, 0);
其结果也是完全一样的。
实验2 使用fcntl函数设置在打开的文件末尾添加内容
fcntl.c
//修改文件描述符的flag属性
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/stat.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
//打开文件
int fd = open(argv[1], O_RDWR);
if(fd<0)
{
perror(&#34;open error&#34;);
return -1;
}
//获得和设置fd的flags属性
int flags = fcntl(fd, F_GETFL, 0);
flags = flags | O_APPEND;
fcntl(fd, F_SETFL, flags);
//写文件
write(fd, &#34;hello world&#34;, strlen(&#34;hello world&#34;));
//关闭文件
close(fd);
return 0;
}结果
[root@ae832bd6d3df IO_C]# make fcntl
cc fcntl.c -o fcntl
[root@ae832bd6d3df IO_C]# head hello.log
test1 ....test ....ni hao hello world
[root@ae832bd6d3df IO_C]# ./fcntl hello.log
[root@ae832bd6d3df IO_C]# head hello.log
test1 ....test ....ni hao hello worldhello world4、统计目录下的文件数量
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
#include <dirent.h>
int main(int argc, char* argv[])
{
int n = checkdir(argv[1]);
printf(&#34;n == [%d]&#34;, n );
}
int checkdir(char *path)
{
//打开目录
DIR *pDir = opendir(path);
if(pDir==NULL)
{
perror(&#34;opendir error&#34;);
return -1;
}
//循环读取目录
int n = 0;
char sFullPath[1024];
struct dirent *pDent = NULL;
while((pDent=readdir(pDir))!=NULL)
{
if(strcmp(pDent->d_name, &#34;.&#34;)==0 || strcmp(pDent->d_name, &#34;..&#34;)==0 )
{
continue;
}
printf(&#34;[%s]--->&#34;, pDent->d_name);
//判断文件类型
switch(pDent->d_type)
{
case DT_DIR:
printf(&#34;目录文件\n&#34;);
memset(sFullPath, 0x00, sizeof(sFullPath));
sprintf(sFullPath, &#34;%s/%s&#34;, path, pDent->d_name); //拼接路径和文件夹名称
n += checkdir(sFullPath);
break;
case DT_REG:
printf(&#34;普通文件\n&#34;);
n++;
break;
case DT_LNK:
printf(&#34;链接文件\n&#34;);
break;
default:
printf(&#34;&#34;);
}
}
closedir(pDir);
return n;
}
和之前的差异就是,多了个循环调用:
memset(sFullPath, 0x00, sizeof(sFullPath));
sprintf(sFullPath, &#34;%s/%s&#34;, path, pDent->d_name);//拼接路径和文件夹名称
n += checkdir(sFullPath); |
|